This java program finds if a number is odd or even. If the number is divisible by 2 then it will be even, otherwise it is odd. We use modulus operator to find remainder in our program.
Output of program:
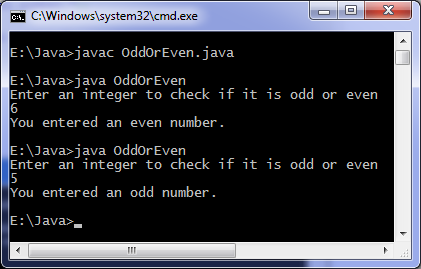
Another method to check odd or even
There are other methods for checking odd/even one such method is using bitwise operator.
Output of program:
Java programming source code
import java.util.Scanner; class OddOrEven { public static void main(String args[]) { int x; System.out.println("Enter an integer to check if it is odd or even "); Scanner in = new Scanner(System.in); x = in.nextInt(); if ( x % 2 == 0 ) System.out.println("You entered an even number."); else System.out.println("You entered an odd number."); } }
Output of program:
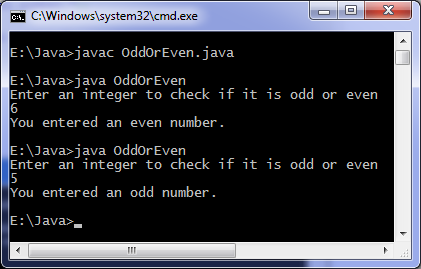
Another method to check odd or even
import java.util.Scanner; class EvenOdd { public static void main(String args[]) { int c; System.out.println("Input an integer"); Scanner in = new Scanner(System.in); c = in.nextInt(); if ( (c/2)*2 == c ) System.out.println("Even"); else System.out.println("Odd"); } }
There are other methods for checking odd/even one such method is using bitwise operator.
import java.util.Scanner; public class EvenOrOdd { public static void main(String args[]) { Scanner obj = new Scanner(System.in); int no; System.out.println("Enter any number to check if it is Even or Odd number:"); no = obj.nextInt(); isOddOrEven(no); } public static void isOddOrEven(int number){ if((number & 1) == 0){ System.out.println("Using bitwise operator: " + number + " is Even number"); } else{ System.out.println("Using bitwise operator: " + number + " is Odd number"); } } }
Output of program:
