import java.util.Scanner;
class Division {
public static void main(String[] args) {
int a, b, result;
Scanner input = new Scanner(System.in);
System.out.println("Input two integers");
a = input.nextInt();
b = input.nextInt();
result = a / b;
System.out.println("Result = " + result);
}
}
Now we compile and execute the above code two times, see the output of program in two cases:
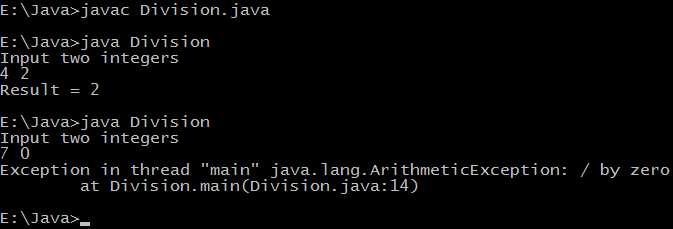
In the second case we are dividing a by zero which is not allowed in mathematics, so a run time error will occur i.e. an exception will occur. If we write programs in this way then they will be terminated abnormally and user who is executing our program or application will not be happy. This occurs because input of user is not valid so we have to take a preventive action and the best thing will be to notify the user that it is not allowed or any other meaningful message which is relevant according to context. You can see the information displayed when exception occurs it includes name of thread, file name, line of code (14 in this case) at which exception occurred, name of exception (ArithmeticException) and it's description('/ by zero'). Note that exceptions don't occur only because of invalid input only there are other reasons which are beyond of programmer control such as stack overflow exception, out of memory exception when an application requires memory larger than what is available.
Java provides a powerful way to handle such exceptions which is known as exception handling. In it we write vulnerable code i.e. code which can throw exception in a separate block called as try block and exception handling code in another block called catch block. Following modified code handles the exception.
Java exception handling example
class Division {
public static void main(String[] args) {
int a, b, result;
Scanner input = new Scanner(System.in);
System.out.println("Input two integers");
a = input.nextInt();
b = input.nextInt();
// try block
try {
result = a / b;
System.out.println("Result = " + result);
}
// catch block
catch (ArithmeticException e) {
System.out.println("Exception caught: Division by zero.");
}
}
}
Whenever an exception is caught corresponding catch block is executed, For example above code catches ArithmeticException only. If some other kind of exception is thrown it will not be caught so it's the programmer work to take care of all exceptions as in our try block we are performing arithmetic so we are capturing only arithmetic exceptions. A simple way to capture any exception is to use an object of Exception class as other classes inherit Exception class, see another example below:
class Exceptions {
public static void main(String[] args) {
String languages[] = { "C", "C++", "Java", "Perl", "Python" };
try {
for (int c = 1; c <= 5; c++) {
System.out.println(languages[c]);
}
}
catch (Exception e) {
System.out.println(e);
}
}
}
Output of program:
C++
Java
Perl
Python
java.lang.ArrayIndexOutOfBoundsException: 5
Here our catch block capture an exception which occurs because we are trying to access an array element which does not exists (languages in this case). Once an exception is thrown control comes out of try block and remaining instructions of try block will not be executed. At compilation time syntax and semantics checking is done and code is not executed on machine so exceptions can only be detected at run time.
Finally block in Java
Finally block is always executed whether an exception is thrown or not.
class Allocate {
public static void main(String[] args) {
try {
long data[] = new long[1000000000];
}
catch (Exception e) {
System.out.println(e);
}
finally {
System.out.println("finally block will execute always.");
}
}
}
Output of program:
finally block will execute always.
Exception in thread "main" java.lang.OutOfMemoryError: Java heap space
at Allocate.main(Allocate.java:5)
Exception occurred because we try to allocate a large amount of memory which is not available. This amount of memory may be available on your system if this is the case try increasing the amount of memory to allocate through the program.