Static methods in Java can be called without creating an object of class. Have you noticed why we write static keyword when defining main it's because program execution begins from main and no object has been created yet. Consider the example below to improve your understanding of static methods.
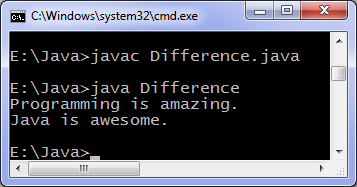
If you wish to call static method of another class then you have to write class name while calling static method as shown in example below.
10 200
Here we are using min and max methods of Math class, min returns minimum of two integers and max returns maximum of two integers. Following will produce an error:
min();
We need to write class name because many classes may have a method with same name which we are calling.
Java static method example program
class Languages { public static void main(String[] args) { display(); } static void display() { System.out.println("Java is my favorite programming language."); } }
Output of program:
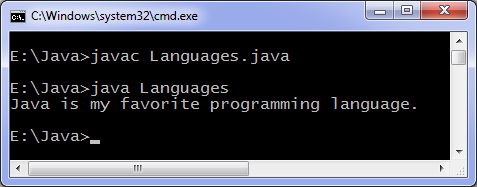
Instance method requires an object of its class to be created before it can be called while static method doesn't require object creation.
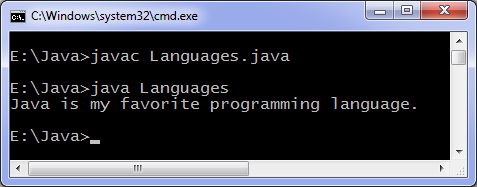
Java static method vs instance method
class Difference { public static void main(String[] args) { display(); //calling without object Difference t = new Difference(); t.show(); //calling using object } static void display() { System.out.println("Programming is amazing."); } void show(){ System.out.println("Java is awesome."); } }Output of code:
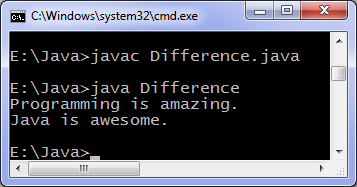
Using static method of another classes
If you wish to call static method of another class then you have to write class name while calling static method as shown in example below.
import java.lang.Math; class Another { public static void main(String[] args) { int result; result = Math.min(10, 20); //calling static method min by writing class name System.out.println(result); System.out.println(Math.max(100, 200)); } }Output of program:
10 200
Here we are using min and max methods of Math class, min returns minimum of two integers and max returns maximum of two integers. Following will produce an error:
min();
We need to write class name because many classes may have a method with same name which we are calling.
0 Comments:
Post a Comment