Given below is the code of java program that adds two numbers which are entered by the user.
Java programming source code
import java.util.Scanner; class AddNumbers { public static void main(String args[]) { int x, y, z; System.out.println("Enter two integers to calculate their sum "); Scanner in = new Scanner(System.in); x = in.nextInt(); y = in.nextInt(); z = x + y; System.out.println("Sum of entered integers = "+z); } }
Output of program:
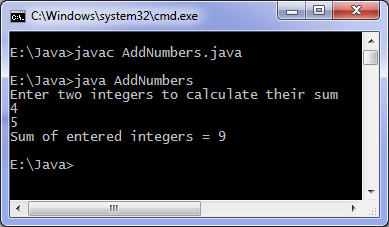
Above code can add only numbers in range of integers(4 bytes), if you wish to add very large numbers then you can use BigInteger class. Code to add very large numbers:
import java.util.Scanner; import java.math.BigInteger; class AddingLargeNumbers { public static void main(String[] args) { String number1, number2; Scanner in = new Scanner(System.in); System.out.println("Enter first large number"); number1 = in.nextLine(); System.out.println("Enter second large number"); number2 = in.nextLine(); BigInteger first = new BigInteger(number1); BigInteger second = new BigInteger(number2); BigInteger sum; sum = first.add(second); System.out.println("Result of addition = " + sum); } }
In our code we create two objects of BigInteger class in java.math package. Input should be digit strings otherwise an exception will be raised, also you cannot simply use '+' operator to add objects of BigInteger class, you have to use add method for addition of two objects.
Output of program:
Enter first large number
11111111111111
Enter second large number
99999999999999
Result of addition = 111111111111110
0 Comments:
Post a Comment